Table Of Content

First of all, we have to make our class constructor private so that we can not create objects from outside of the class using the new keyword. In this article, I will discuss the Singleton Design Pattern in C# with Examples. Please read our previous article discussing Shallow Copy and Deep Copy in C# with Examples. The Singleton Design Pattern in C# falls under the Creational Pattern Category.
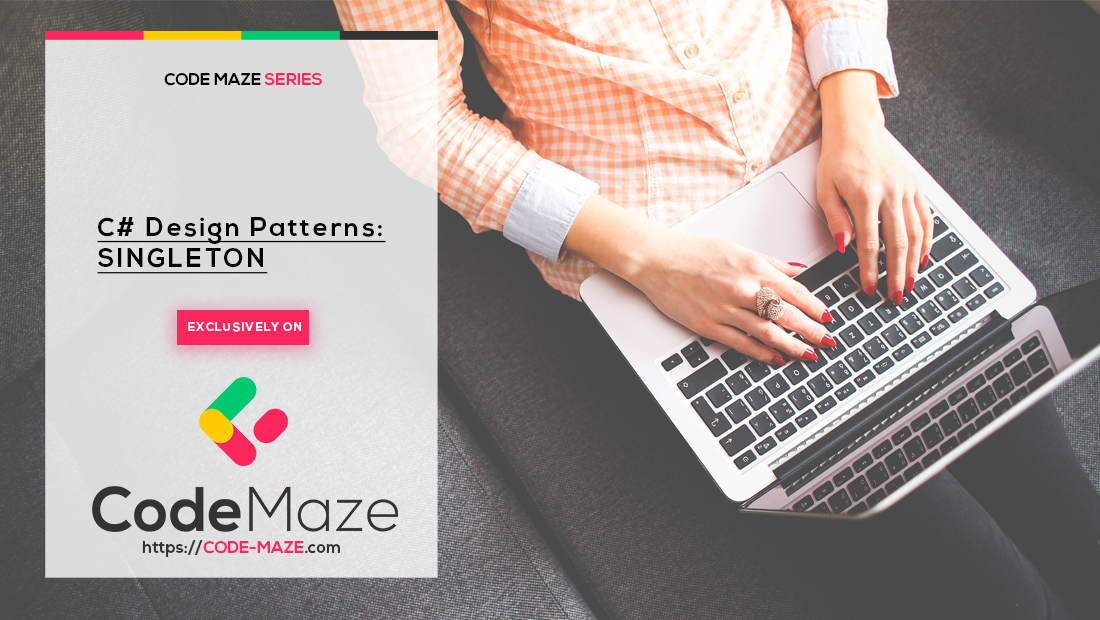
Creational Software Design Patterns in C++
And also, we can’t inherit this class into another class because of a private constructor. In this pattern, we have to restrict creating the instance if it is already created and use the existing one. The VoteMachine singleton class will work perfectly in the synchronous calls where each user will register their vote one by one. The following is the basic structure of the singleton class in C#. In the next article, I will discuss Why we Need to use the Sealed Keyword in the Singleton Class, as we already have a private constructor. In this article, I explain the basic concepts of the Singleton Design Pattern in C# with Examples.
Use Case of Pattern Singleton Method
The right way to implement Singleton is by dependency injection, So instead of directly depending on a singleton, you might want to consider it depending on an abstraction(e.g. an interface). I would also encourage you to use synchronization primitives(like a mutex, semaphores, etc) to control access. You must know that Singleton instance doesn't need to be manually deleted by us. We need a single object of it throughout the whole program, so at the end of program execution, it will be automatically deallocated.
Software Design Pattern in Development
In software engineering, the singleton pattern is a design pattern that is used to restrict instantiation of a class to one object. This is useful when exactly one object is needed to coordinate actions across the system. The concept is sometimes generalized to systems that operate more efficiently when only one object exists, or that restrict the instantiation to a certain number of objects (say, five). Some consider it an anti-pattern, judging that it is overused, introduces unnecessary limitations in situations where a sole instance of a class is not actually required, and introduces global state into an application. So, both threads will enter into the if condition and create new instances.
C# Design Pattern: Singleton
This method caches the first created object and returns it in all subsequent calls. A small program repeatedly calling the print method of the logger instance concurrently is built to test our singleton Logger. Logger() is the Logger class private constructor, creating the logger object. In software engineering, design patterns are software bricks, recognized as good practice, and addressing common design issues. Go to the Program.cs class and create two methods named LogEmployeeRequest and LogManagersRequest and move the logging code for both the instances to these methods as shown below. A lot of theory covered, now let's practically implement the singleton pattern.
Can You Even __init__.py?. 3 things __init__.py can do for you by Louis Chan Feb, 2024 - Towards Data Science
Can You Even __init__.py?. 3 things __init__.py can do for you by Louis Chan Feb, 2024.
Posted: Thu, 29 Feb 2024 08:00:00 GMT [source]
If we run this program, the result will be exactly same as the previous example. So, why this way is more complete and secure than the first example? Because in the “getInterestRate” method, we check if the “iRate” object is created, and if it is not, we create the “iRate” object here (13th line).
The class can ensure that no other instance can be created (by intercepting requests to create new objects), and it can provide a way to access the instance. We have declared the obj volatile which ensures that multiple threads offer the obj variable correctly when it is being initialized to the Singleton instance. This method drastically reduces the overhead of calling the synchronized method every time.
In one of my implementations, I called queue_ref() in AppDelegate.m (main.c would work, too). That way, queue will be initialized before any other calling object attempts to access it. Returning a value from a variable is much faster than calling a function, and then checking the value of the variable before returning it. I provide an explicit init() and term() methods for all the singletons and call them inside main.
2. Private Constructor:
After getting the design patterns, It is very easy to implement and build project architecture in real-time scenarios. The design patterns make the applications Reliable, Scalable, and easily Maintainable. Implementations of the singleton pattern ensure that only one instance of the singleton class ever exists and typically provide global access to that instance. Although there can be many printers in a system, there should be only one printer spooler. How do we ensure that a class has only one instance and that the instance is easily accessible? A global variable makes an object accessible, but it doesn’t keep you from instantiating multiple objects.
Now, let's test the above VoteMachine class in the multi-threaded scenario, as shown below. The following demonstrates the testing of the VoteMachine class in the multi-threaded environment using the Parallel class. Clients may not even realize that they’re working with the same object all the time. In this article, learn what a Singleton Design Pattern is and how to implement a Singleton Pattern in C#.
One of the well-known "Gang of Four" design patterns, which describes how to solve recurring problems in object-oriented software,[1] the pattern is useful when exactly one object is needed to coordinate actions across a system. The Singleton pattern or pattern singleton incorporates a private constructor, which serves as a barricade against external attempts to create instances of the Singleton class. This ensures that the class has control over its instantiation process. Singleton Design pattern is very useful for the logger implementation in our application and this is the famous design pattern.
No comments:
Post a Comment